UIFigure 내에 UIAxes를 만들고 마우스가 UIAxes 위로 이동할 때 현재 좌표를 출력하는 간단한 UI를 만들어 봅시다.
먼저, axes 하나와 좌표를 표시할 label 하나를 생성합니다.
1 2 3 4 5 6 7 8 9 10
| fig = uifigure; ax = uiaxes(fig);
ax.Position(2) = ax.Position(2) + 40; ax.Position(3) = fig.Position(3) - 20; ax.Position(4) = fig.Position(4) - 50;
lb = uilabel(fig); lb.Position = [20, 5, 100, 50]; lb.Text = '(0.00, 0.00)';
|
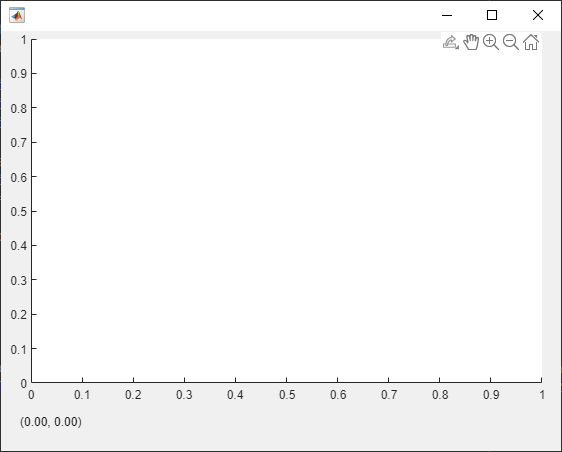
UIFigure 객체의 WindowButtonMotionFcn 속성을 사용합니다.
이 속성은 UIFigure 내에서 마우스가 움직일 때 마다 동작하는 콜백 함수를 설정합니다.
btnMotionFcn 이라는 이름의 콜백 함수를 입력합니다.
1
| fig.WindowButtonMotionFcn = @btnMotionFcn;
|
먼저, 콜백 함수 내에서 마우스의 현재 위치를 보여주는 코드를 작성해 보겠습니다.
1 2 3
| function btnMotionFcn(src, ~) disp(src.CurrentPoint); end
|
실행한 뒤 figure 위에서 마우스를 움직여보면 현재 좌표가 출력되는 것을 확인할 수 있습니다.
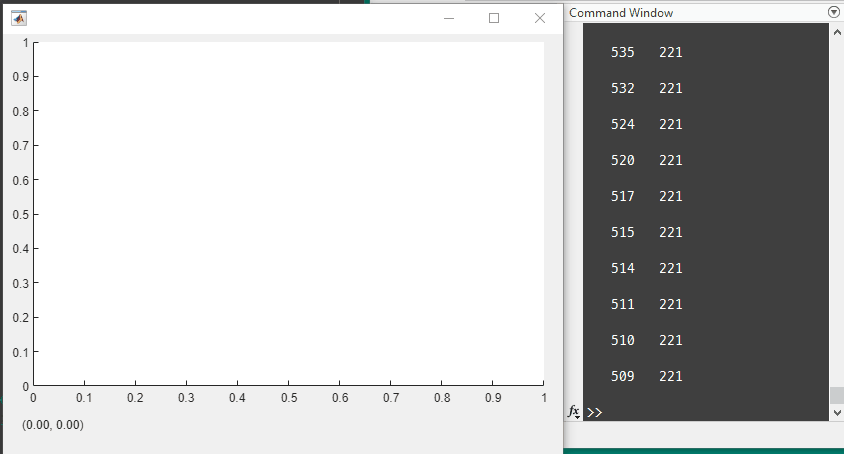
이제 기본적인 틀은 완성 되었으므로 목표대로 axes의 좌표를 가져와서 label에 출력하는 코드를 작성합니다.
이 때, axes와 label 객체는 findobj 함수를 통해 가져오고 axes 외부를 벗어나는 경우 좌표를 출력하지 않는 조건문을 추가합니다.
1 2 3 4 5 6 7 8
| function btnMotionFcn(src, ~) ax = findobj(src, 'Type', 'Axes'); lb = findobj(src, 'Type', 'UILabel');
if ax.CurrentPoint(1, 3) == 1 && ax.CurrentPoint(1, 1) >= ax.XLim(1) && ax.CurrentPoint(1, 1) <= ax.XLim(2) lb.Text = sprintf('(%.2f, %.2f)', ax.CurrentPoint(1, 1), ax.CurrentPoint(1, 2)); end end
|
이제 마우스를 움직여보면 axes 내부에서만 좌표가 표시되는 것을 확인할 수 있습니다.
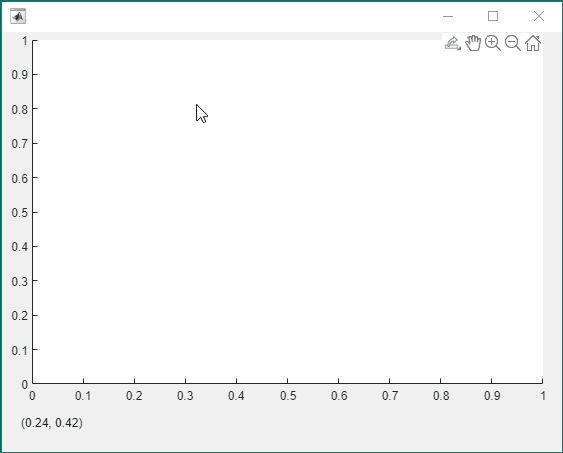
임의의 데이터를 plot하고, mouse pointer 옵션을 약간 변경해 봅시다.
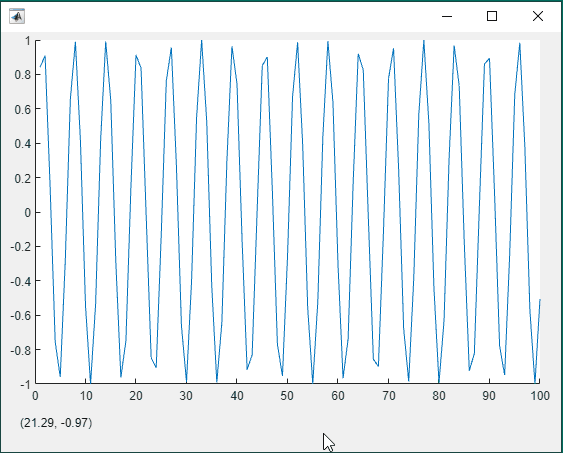
전체 소스코드
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| fig = uifigure; ax = uiaxes(fig);
ax.Position(2) = ax.Position(2) + 40; ax.Position(3) = fig.Position(3) - 20; ax.Position(4) = fig.Position(4) - 50;
lb = uilabel(fig); lb.Position = [20, 5, 100, 50]; lb.Text = '(0.00, 0.00)';
fig.WindowButtonMotionFcn = @btnMotionFcn; x = 1:100; y = sin(x); plot(ax, x, y);
function btnMotionFcn(src, ~)
src.Pointer = 'arrow'; ax = findobj(src, 'Type', 'Axes'); lb = findobj(src, 'Type', 'UILabel');
if ax.CurrentPoint(1, 3) == 1 && ax.CurrentPoint(1, 1) >= ax.XLim(1) && ax.CurrentPoint(1, 1) <= ax.XLim(2) lb.Text = sprintf('(%.2f, %.2f)', ax.CurrentPoint(1, 1), ax.CurrentPoint(1, 2)); src.Pointer = 'crosshair'; end
end
|